Android基础:Fragment的使用技巧
来源:|发布时间:2017-04-28|浏览量:
一、静态使用
Fragment,即碎片,可以理解为是为了适配大屏幕的安卓设备而生的。Fragment出现后,深受安卓开发者的喜爱。Fragment很好用但也不容易用。下面我来来细细解说Android中的Fragment。
1、Fragment产生的缘由
运行Android的设备繁多,屏幕大小更是多种多样。针对不同屏幕尺寸,通常情况下,开发者都是先针对手机开发一套源代码,然后拷贝一份,修改布局以适应大屏幕设备,或平板,电视等。为了决解这样的麻烦,Google推出了Fragment。你可以把Fragment当成Activity的一个界面的一个组成部分,甚至Activity的界面可以完全有不同的Fragment组成,Fragment拥有自己的生命周期和接收、处理用户的事件,这样就不必在Activity写一堆控件的事件处理的代码了。更为重要的是,你可以动态的添加、替换和移除某个Fragment。
2、Fragment的生命周期
Fragment必须是依存与Activity而存在的,因此Activity的生命周期会直接影响到Fragment的生命周期;很好的说明了两者生命周期的关系:
可以看到Fragment比Activity多了几个额外的生命周期回调方法: onAttach(Activity) 当Fragment与Activity发生关联时调用。 onCreateView(LayoutInflater, ViewGroup,Bundle) 创建该Fragment的视图 onActivityCreated(Bundle) 当Activity的onCreate方法返回时调用 onDestoryView() 与onCreateView想对应,当该Fragment的视图被移除时调用 onDetach() 与onAttach相对应,当Fragment与Activity关联被取消时调用 注意:除了onCreateView,其他的所有方法如果你重写了,必须调用父类对于该方法的实现,
3、静态的使用Fragment
这是使用Fragment最简单的一种方式,把Fragment当成普通的控件,直接写在Activity的布局文件中。步骤:
1、继承Fragment,重写onCreateView决定Fragemnt的布局
2、在Activity中声明此Fragment,就当和普通的View一样
下面展示一个例子(我使用2个Fragment作为Activity的布局,一个Fragment用于标题布局,一个Fragment用于内容布局):
TitleFragment的布局文件:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" android:layout_width="match_parent" android:background="@android:color/darker_gray" android:layout_height="45dp"> <ImageButton android:id="@+id/im_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@android:drawable/star_on" /> <TextView android:textSize="25sp" android:textColor="@android:color/holo_red_dark" android:gravity="center" android:text="Fragment制作标题栏" android:layout_width="match_parent" android:layout_height="match_parent" /> </RelativeLayout> |
ContentFragment布局文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:gravity="center" android:layout_height="match_parent"> <TextView android:gravity="center" android:textSize="25sp" android:text="Fragment当作主面板" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout> |
MainActivity布局文件
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <fragment android:id="@+id/fg_title" android:layout_width="match_parent" android:layout_height="45dp" android:name="app.linfeng.com.myapplication.TitleFragment" /> <fragment android:layout_below="@id/fg_title" android:id="@+id/fg_content" android:layout_width="match_parent" android:layout_height="match_parent" android:name="app.linfeng.com.myapplication.ContentFragment" /> </RelativeLayout> |
TitleFragment源代码
package app.linfeng.com.myapplication; import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ImageButton; import android.widget.Toast; public class TitleFragment extends Fragment { PRivate ImageButton im_button; @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.title_fragment_layout,container,false); im_button = (ImageButton) view.findViewById(R.id.im_button); im_button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(getContext(),"业务逻辑写在Fragment上,Activity是不是很整洁了?",Toast.LENGTH_SHORT).show(); } }); return view; } } |
ContentFragment源代码
package app.linfeng.com.myapplication; import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class ContentFragment extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View view = inflater.inflate(R.layout.content_fragment_layout,container,false); return view; } } |
MainActivity源代码
package app.linfeng.com.myapplication; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; /** * 因为Android Studio新建Activity是自动继承AppCompatActivity,所以我也没有改成 * Activity,这个和本案例没有关系哈。 */ public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // requestWindowFeature(Window.FEATURE_NO_TITLE); //因为继承的是AppCompatActivity,所以requestWindowFeature()失效了 getSupportActionBar().hide(); setContentView(R.layout.activity_main); } } |
静态使用Fragment其实就是把Fragment当成普通的View一样声明在Activity的布局文件中,然后所有控件的事件处理等代码都由各自的Fragment去处理,瞬间觉得Activity好干净有木有~~代码的可读性、复用性以及可维护性是不是瞬间提升了
二、动态添加
package com.itheima.dyncfragment; import android.os.Bundle; import android.app.Activity; import android.app.FragmentManager; import android.app.FragmentTransaction; import android.view.Menu; import android.view.WindowManager; public class MainActivity extends Activity { @SuppressWarnings("deprecation") @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //[1]获取手机的分辨率 WindowManager wm = (WindowManager) getSystemService(WINDOW_SERVICE); int width = wm.getDefaultDisplay().getWidth(); int height = wm.getDefaultDisplay().getHeight(); //[2]判断横竖屏 //[3]获取Fragment的管理者 通过上下文直接获取 FragmentManager fragmentManager = getFragmentManager(); FragmentTransaction beginTransaction = fragmentManager.beginTransaction(); //开启事物 if(height > width){ //说明是竖屏 加载一个Fragment android.R.id.content //代表当前手机的窗体 beginTransaction.replace(android.R.id.content, new Fragment1()); }else { //说明是横屏 加载一个Fragment beginTransaction.replace(android.R.id.content, new Fragment2()); } //[4]最后一步 记得comment beginTransaction.commit(); } } |
下一篇:谷歌I/O开发者大会来袭,Android O正式版将登场
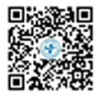
扫码关注微信公众号了解更多详情
跟技术大咖,专业导师一起交流学习
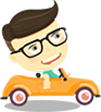